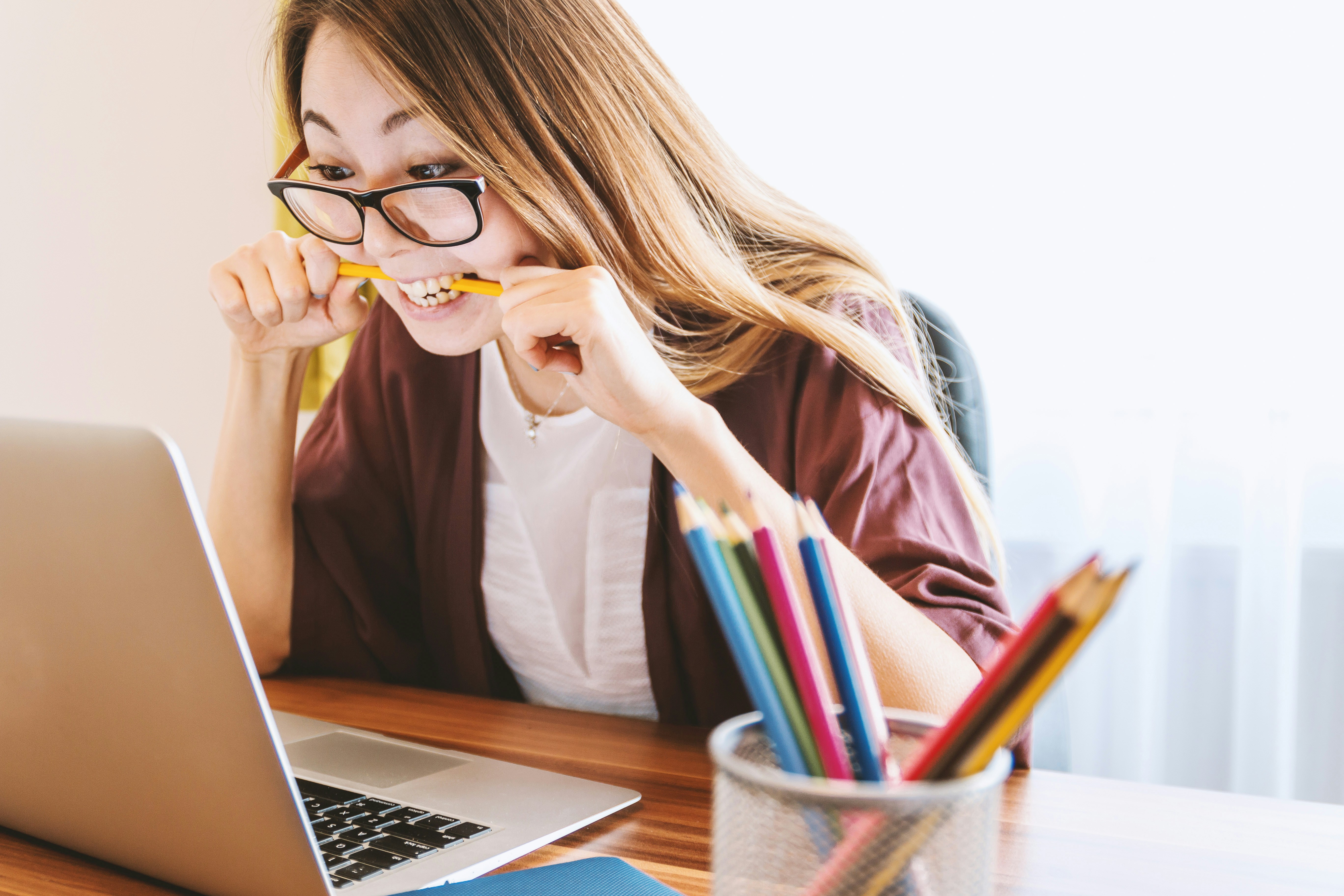
Get started Programming
Welcome to the world of programming!
Brief overview
Whether you’re looking to build a tech career, automate tasks, or simply explore a new hobby, starting your programming journey can be both exciting and overwhelming. Here are some essential tips to help you kickstart your learning path effectively.
### My background programming
I have been working in IT for over 20 years, during this time more than 10 years I worked as a Java developer then moved to a Consultant field. I didn’t stop developing just became less often as I needed to do more troubleshooting, and configurations, implement foundations for projects, pilots, PoC and eventually when I get involved in long-term project to implement the end to end solution.
So I am using my experience and how I learned how to program to help you as well on your journey.
You may have watched tons of videos on YouTube to help you start programming, and a lot of online courses but still you can get a simple task implemented/coded on your own. So you come to the right place.
First thing let’s take one step back before you jump to choose that you need to learn Python, Java, GO, C#, C++, etc, what are the fundamentals to get started programming
Programming “Way of Thinking”
I asked a lot of friends in the field how was their experience learning programming.
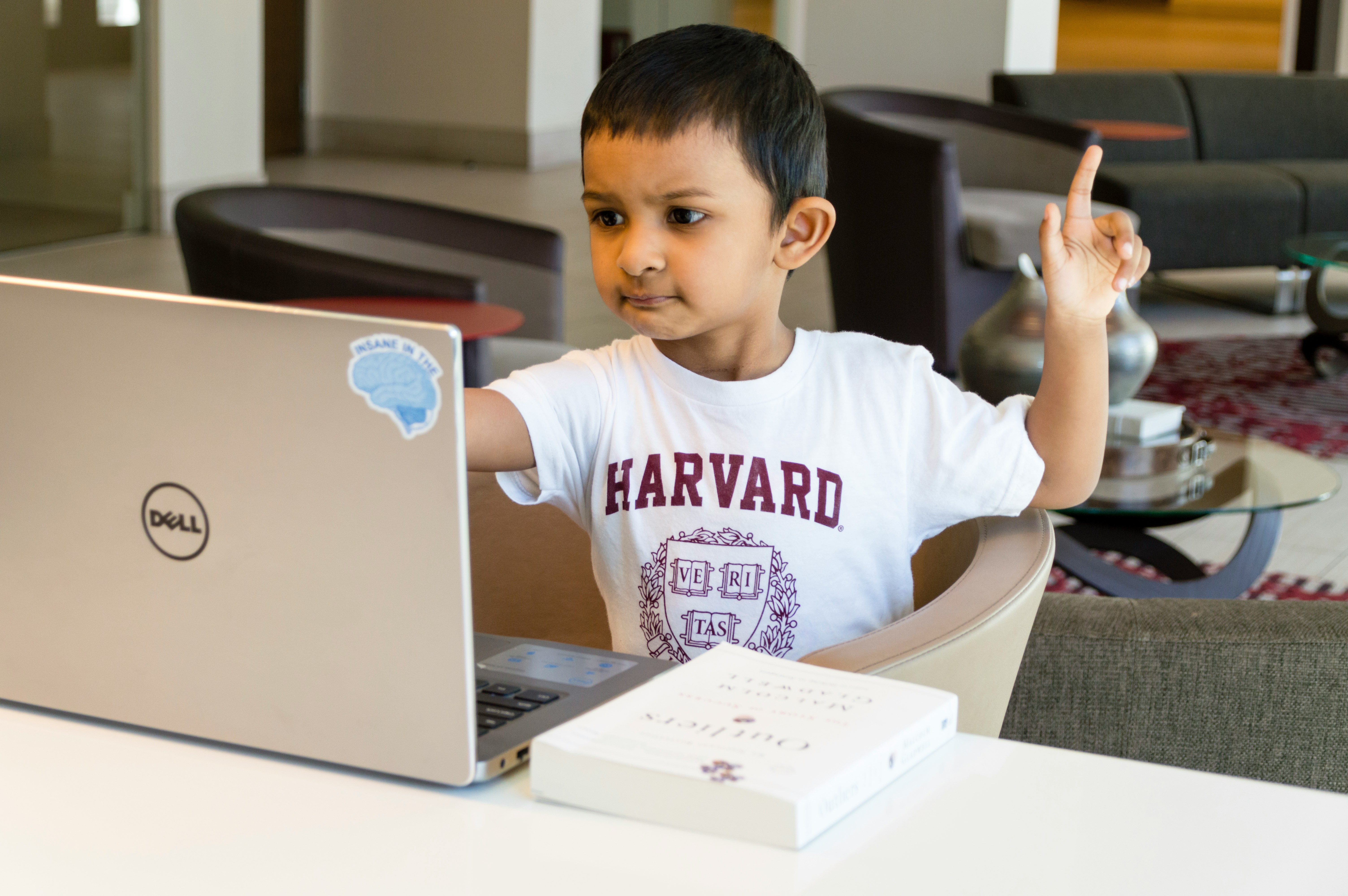
Every time I got the same answer, suddenly they felt like a pin came out of the head something clicked and programming started to make more sense. Their whole way of thinking, looking to use code to resolve a problem changes, and it becomes natural.
Just like my friends and myself, you might feel frustrated in the beginning. But once everything clicks, you’ll see programming as a whole new way of thinking. So don’t panic if you have not clicked yet, it’s the reason they called programming a language.
Programming is a language that communicates with computers, similar to learning any new spoken language. Sometimes you keep absorbing information about it, but you can’t ‘speak’ it fluently until you’ve practised enough.
You know, it’s like when people tell you that to speak French, English or any language you need to think in that language so it starts to come naturally, after you get your head around with program thinking will start to make sense. That is when your brain decodes coding.
Ok, so how to think in a way that programming will come naturally to you?
It’s a lot of different answers to this I will give you what works for me. Right, I did a lot of problem-solving exercises and trained my mind to a more logical programming paradigm.
Training to think in a logical sequence.
How to do that?
Simply you need to think in a sequence of tasks that when performed will end with the same results, for example:
Let’s try this, exercise here to help to change your mindset, remember programming is like any other mental exercise that you need to practice a lot to strengthen your mental “muscles”.
If you push a pen out from your table, what going to happen?
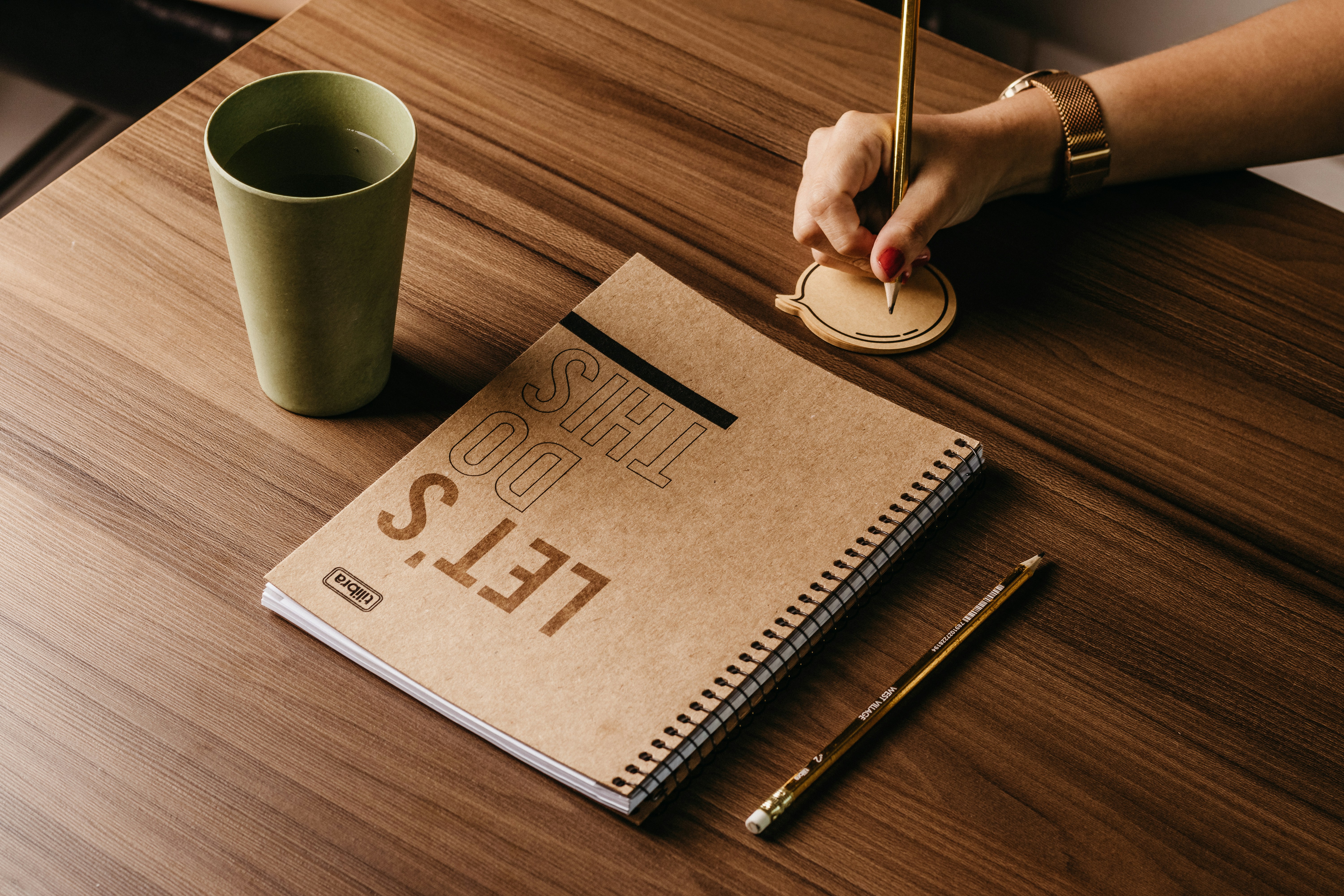
It will soon fall out on the floor.
Then you can ask yourself every time you push that pen out of the table will always have the same result?
Is there any case that the pen will fall into the ceiling? Of course not
Let’s think In another situation, now.
If I open the window would that make rain, is there any logical reason that doing a task like opening the window will result in rainfall?
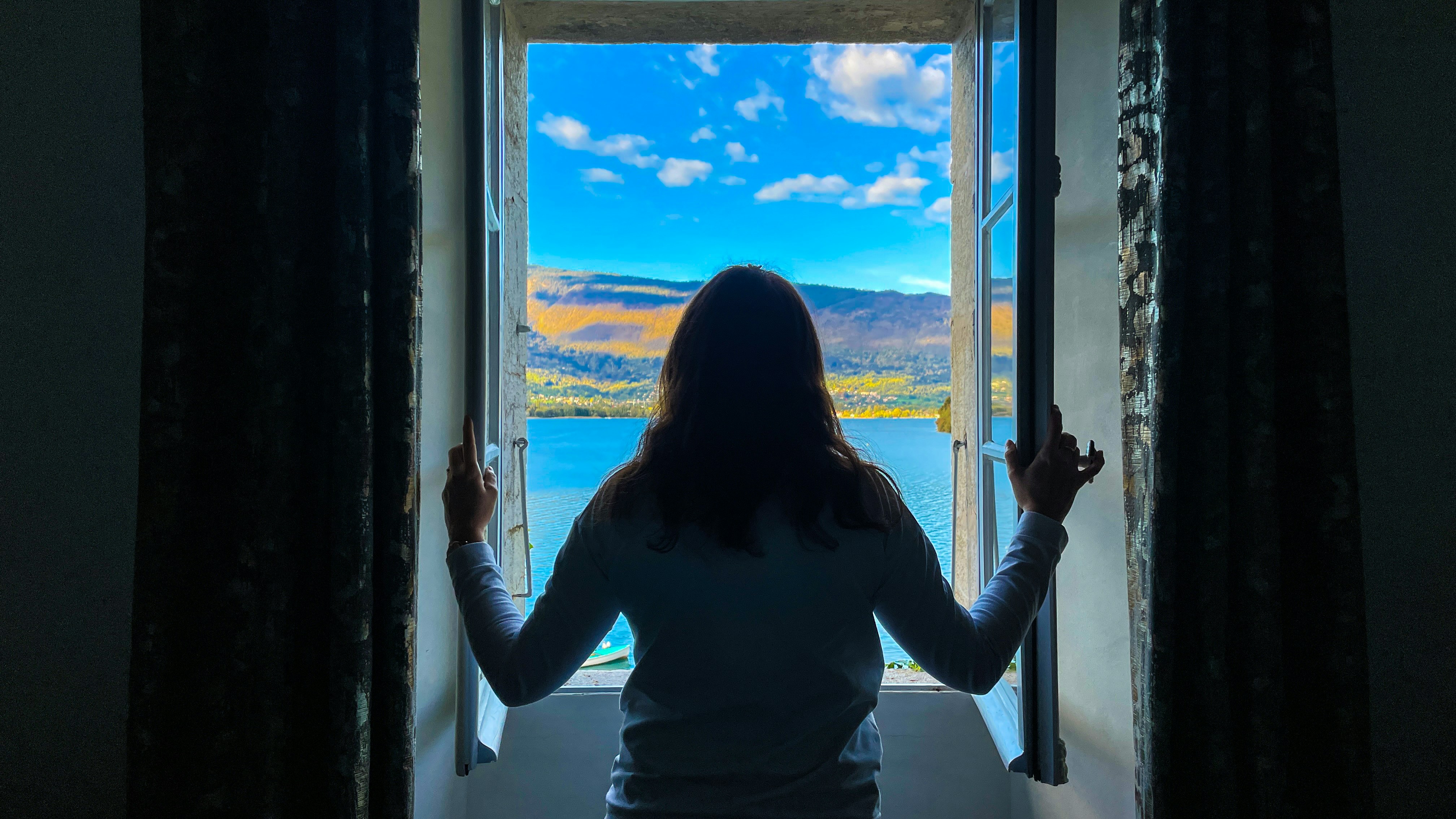
It’s a logical end for every time you push the pen from the table but there’s no logical connection between opening a window and raining.
So the tasks need to follow a sequence that will be logical and will end up producing the same outcome.
Programming will follow the same logic struct, when you execute some sequence of tasks will have an expected result.
Right now that we have established this, let’s think about a cake recipe, I love carrot cake and whether you cooked it or not you know that if you don’t follow the recipe your cake will be s* or just not good enough or just different.
Let’s think now about what a carrot cake needs, sugar, flour, eggs, oil, etc.
Right, you have the ingredients and items to put and mix.
Items:
- 1 cup brown sugar.
- 2 cups all-purpose flour.
- 4 large eggs.
- 265g carrots.
- Etc.
Think now in the step-by-step process to make it.
Steps:
- Preheat the Oven
- Mix the Dry Ingredients
- Add the eggs
- Combine wet and dry and mix everything
- Bake the cake
The ingredients in this scenario are the data, so that is the information you will provide to your program.
Your program needs to store that information somewhere to access it later, that is where you create some called ‘variables’. Variables are where it will set external information provided by the user to be used within the steps you will perform in your code.
The steps to prepare that cake are the steps to perform in your program, line of codes like mix these two values or recipe items or validate if the amount of sugar or data is correct.
Something like if carrot grams are less than 265g then add more, if is more then remove it until is 256g, now add oil, and sugar and mix it 10 times.
Then after that put the cake to bake for 20 min.
Why doing that? Because programming is a logic set of sequences in logical form, following a sequence of tasks to resolve a problem in a specific domain.
Understanding Key Programming Concepts: Conditional Statements, Loops, and Functions
Programming is to apply those sequences of tasks to resolve a problem.
In programming, these steps translate into the lines of code you will write.
Then any tasks that need to be repeated are managed with loop structures like for and while, things like mixing these ingredients 10 times or mixing while the mixture still not soft enough.
There are specific pieces of code for tasks like mixing ingredients or baking the cake. When you notice these are tasks repeated every time, regardless of whether it’s a carrot or chocolate cake for example you will always need to back. Then you can wrap them in a function or method. This allows you to reuse the same code throughout the program.
These are known as syntax structures, which allow us to check and validate data, repeat tasks with loops, and handle other operations. Common examples include if
, else
, when
, and for
, which you’ve likely heard of before.
Every programming language has these types of structures, though the syntax may vary slightly or significantly from one language to another.
Introduction to Variables: Capturing and Displaying User Input in Programming
Let’s try now with a more practical problem.
You can practice this on a piece of paper or a drawing tool on your laptop.
Let’s say now that you have to give instructions to a computer to capture the person’s name and then show it on the screen with the message Welcome and the person’s name.
When someone tells us this information you memorize it, in programming you need to tell the computer to save it in its memory so it can use it later on.
So, the information you need to store that information in memory for the program to access it later is called variables.
The information will vary every time a new user uses the program it needs to be stored again in the same variable in your code to have a different result.
You can have a variable name
to save any person’s name the user types, the command to save it is normally used with the equal sign =
.
name = ‘Paulo’
Let’s use pseudo-programming language to explain this.
name = NULL
name = INPUT “Please type your name:”
DISPLAY "Welcome, " + name + "!"
Let’s break down these tasks.
name = NULL
This means we’re creating an empty “box” (called a variable) named name. Right now, there’s nothing inside the box—it’s empty. In programming, we use NULL to say “This has no value yet.” This creates a space in the computer memory with an address that every type you set a new value will override the previous value.
name = INPUT “Please type your name:”
Here, you’re asking the computer to show a message to the user: “Please type your name.”
The user types in their name, and the computer stores that name inside the “name” box.
So now, instead of being empty, the name box holds the name the user typed. For example, if the user types “Paulo,” the name box will now contain “Paulo.”
Then when you want to display it in the console you do the following command
DISPLAY "Welcome, " + name + "!"
Now, the computer will show a message that says “Welcome” followed by the name from the name box, and an exclamation point.
If the user typed “Paulo,” the computer will show: “Welcome, Paulo!”
This is done by combining the words “Welcome,” the name from the box (e.g., “Paulo”), and an exclamation point. This is to put words/text together then in programming they will give a fancy name called concatenate string.
So if you run this code again and type a different name the variable called name will receive this new user name and display it like before.
Python
Let’s see how that would be in a Python syntax.
# Welcome sample
name = input("Please type your name:")
print("Welcome "+name+"!")
This Python code takes user input and displays a personalized welcome message. Here’s a breakdown of how it works:
Explanation:
- Comment (
# Welcome sample
):- The line starting with
#
is a comment. Comments are ignored by the Python interpreter and are meant to explain the code to other people. In this case, it’s simply noting that this is a “Welcome sample.” It’s normally used to document your code.
- The line starting with
- User Input (
name = input("Please type your name:")
):input("Please type your name:")
: This is a built-in Python function that prompts the user to type something. The string inside the parentheses ("Please type your name:"
) is what the user will see on the screen as the prompt.- The user then types their name, and whatever they type is captured and stored in the variable
name
.
For example, if the user types “Paulo,” the variable
name
will now hold the value"Paulo"
. - Display Message (
print("Welcome "+name+"!")
):print()
: This function outputs whatever is inside the parentheses to the screen."Welcome "
: This is a string (text) that the function will print.+name+
: The+
symbol concatenates (joins) the string"Welcome "
with the value stored in the variablename
. So ifname
is"Paulo"
, the result is"Welcome Paulo"
."!"
: Another string is added at the end of the message to complete the sentence.
Final Output: If the user enters “Paulo,” the program will print:
Welcome Paulo!
Summary:
- The code first asks the user for their name.
- It then prints a personalized message like “Welcome Paulo!” depending on the input given.
You can try adding an extra variable to store the person’s age and with if else check if the person is older than 18 if yes print “adult discount available for you” else “discount for kids menu available for you”
Python Examples
You want to check Python examples like this one and more, you can explore my repo here.
For me what helped was to understand what was going on behind the scenes the other thing was problem-solving exercises.
Problem Solving Examples
You can explore problem-solving exercises here.
This page was last update at 2025-01-16 15:51